@: It's a tutorial, not live code. We also define a variadic function called getSum() that takes in an array of functions that are of type myMultiplier. defined operators. We can pass optional numeric parameters to a variadic function. These input values provide extra information to a function and they are totally optional. Was looking to see if optional parameters or alternatively parameter default values could be done so this is possible; func (n *Note) save(extension string = ".txt") { } making ".txt" the default yet changeable extension of a file. NewUint8 creates an optional.Uint8 from a uint8. Get returns the int value or an error if not present. In programming, a generic type is a type that can be used in conjunction with multiple other types. Get returns the int32 value or an error if not present. Zero number of parameters passed, Start executing
Golang Buffered Channel Tutorial [Practical Examples], How to set CPU Affinity in Golang? https://plus.google.com/107537752159279043170/posts/8hMjHhmyNk2. You also updated the AddCard and RandomCard methods to either accept a generic argument or return a generic value. You can almost think of these type parameters as similar to the parameters youd include in a function. In the function body, we check the length of the names variable and iterate over the parameters if the length is more than one else we print a statement indicating that there are no parameters passed. Totally agree.Sometimes putting nil as parameter can be much simpler than additional changes. Thus, a string cannot be nil.
It would be nice to have a function that does not force us to pass parameters. It specifies the path of the file or directory you want to check. Get returns the bool value or an error if not present. Get returns the int64 value or an error if not present. All rights reserved. Luke 23:44-48. Per the Go for C++ programmers docs. [SOLVED]. What are the use(s) for struct tags in Go? Commentdocument.getElementById("comment").setAttribute( "id", "a64dabd8bbd25a9cd9034dcb51244aaf" );document.getElementById("gd19b63e6e").setAttribute( "id", "comment" ); Save my name and email in this browser for the next time I comment. We know what knowledge developers need in order to be productive and efficient when writing software in Go. Go gives you some nice flexibility when it comes to returning values from a function. Using the Deck this way will work, but can also result in errors if a value other than *PlayingCard is added to the Deck. OrElse returns the byte value or a default value if the value is not present. The ellipsis indicates that the function can b Get returns the uint8 value or an error if not present. Amending Taxes To 'Cheat' Student Loan IBR Payments? If my articles on GoLinuxCloud has helped you, kindly consider buying me a coffee as a token of appreciation. Added a functional example to show functionality versus the sample. . In a function return statement is used to return multiple values. Once you added the TradingCard type, you created the NewTradingCardDeck constructor to create and populate the deck with trading cards. Finally, you created a generic printCard function that could print the name of any Card value using the Name method. This library deals with nil as same as None[T]. When you updated Decks RandomCard method to return C and updated NewPlayingCardDeck to return *Deck[*PlayingCard], it changed RandomCard to return a *PlayingCard value instead of interface{}. MustGet returns the uint8 value or panics if not present. Modules with tagged versions give importers more predictable builds. A lot of code written in Go can work well using just the functionality interfaces provide. In the function signature, the eclipse is preceded with a string type. In this Card interface, youre saying that for something to be considered a Card, it must implement the fmt.Stringer type (it must have the String method your cards already have), and it must also have a Name method that returns a string value. For any other feedbacks or questions you can either use the comments section or contact me form. Not the answer you're looking for? MustGet returns the uint value or panics if not present. Wed like to help. Plagiarism flag and moderator tooling has launched to Stack Overflow! This article will explore optional parameters in Go using variadic functions. Now, update your Deck so it only allows Card types to be used for C: Before this update, you had C any for the type restriction (known as the type constraint), which isnt much of a restriction. The goal for Golang-WASM is to eventually implement a comprehensive section of the DOM-API that will be able to be used in a OrElse returns the rune value or a default value if the value is not present. Other languages call this discrimated union if you include a special field which denote the field being used. This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository. It would be great if structs could have default values here; anything the user omits is defaulted to the nil value for that type, which may or may not be a suitable default argument to the function. The syntax of the multi-return function is shown below: The return types and values can be declared in multiple ways as we will see. The null types from sql such as NullString are convenient. Matching only by name and requiring Connect and share knowledge within a single location that is structured and easy to search. (*foo.type)" if the parameters have non-uniform types. Synopsis some := optional. Once you have all your changes saved, you can run your program using go run with main.go, the name of the file to run: In the output of your program, you should see a card randomly chosen from the deck, as well as the card suit and rank: Since the card is drawn randomly from the deck, your output will likely be different from the output shown above, but you should see similar output. John
In Go values that are returned from functions are passed by value. Go gives you some nice flexibility when it comes to returning values from a function. Here is a simple example of returning two values from a function: The function ReturnId returns a value of type integer and of type error. This is something very common that is done in Go. Get returns the string value or an error if not present. She also enjoys learning and tinkering with new technologies. To declare a variadic function, the type of the final parameter is preceded by an ellipsis . You then used the Deck and PlayingCards to choose a random card from the deck and print out information about that card. And this also supports omitempty option for JSON unmarshaling. This is where generic types get their name. Working on improving health and education, reducing inequality, and spurring economic growth? Structs are composed of multiple key/value pairs in which the key is the structs type and the value is the value stored in the struct. by Mark McGranaghan and Eli Bendersky | source | license. Doe
does lili bank work with zelle; guymon, ok jail inmate search Get returns the float32 value or an error if not present. golang recover return value syntax go 12,665 It is a type assertion which check if the error recovered is of a certain type. As a statically-checked type system, Gos compiler will check these type rules when it compiles the program instead of while the program is running. Lastly, update your main function to create a new Deck of *TradingCards, draw a random card with RandomCard, and print out the cards information: In this last update, you create a new trading card deck using NewTradingCardDeck and store it in tradingDeck. I can't find an equally clear statement that optional parameters are unsupported, but they are not supported either. it enables the default use case to be its simplest: no arguments at all! Go has a feature where if you initialize a variable but do not use it, Go compiler will give errors. When we are returning multiple-type of the value we can declare it like this. This feature is used often in idiomatic Go, for example and more detailed examples are here: ./examples_test.go. A function in Go can return multiple values. Get returns the complex128 value or an error if not present. 5.5
When RandomCard returns *PlayingCard, it means the type of playingCard is also *PlayingCard instead of interface{} and you can access the Suit or Rank fields right away. No one will use interface{} instead of already existing object and original code doesnt have a problem that should be solved with generics. In addition, by saying the instance of your Deck can only contain *PlayingCard, theres no possibility for a value other than *PlayingCard being added to the cards slice. MustGet returns the float64 value or panics if not present. Then, you updated the card function parameter to use the C placeholder type instead of the original interface{} type. @burfl yeah, except the notion of "zero value" is absolutely useless for int/float/string types, because those values are meaningful and so you can't tell the difference if the value was omitted from the struct or if zero value was passed intentionally. . NewInt creates an optional.Int from a int. 2Goroutines goroutine goroutine: goroutine () NewUintptr creates an optional.Uintptr from a uintptr. This package is not in the latest version of its module. Here is an example showing just that. In the case of your Deck type, you only need one type parameter, named C, to represent the type of the cards in your deck. Sane defaults means less code, less code means more maintainability. There was a problem preparing your codespace, please try again. A Computer Science portal for geeks. You do this by referencing your Deck type as you normally would, whether its Deck or a reference like *Deck, and then providing the type that should replace C by using the same square brackets you used when initially declaring the type parameters. NewComplex64 creates an optional.Complex64 from a complex64. In the preceding example, we have defined a variadic function that takes string optional parameters. In this section, you will create a new printCard generic function, and use that function to print out the name of the card provided. Go supports this feature internally. Here, the Return_Type is optional and it contains the types of values that function returns. What exactly did former Taiwan president Ma say in his "strikingly political speech" in Nanjing? Printf ( "%v\n", some. Basically, it would be better to provide them as the methods, but currently, it compromises with the limitation. If it return false means the set if not empty. 3 ==> 3.000000, Start executing
It can also be used as a tool to generate option type wrappers around your own types. After that, you created a new TradingCard type to represent a different type of card your Deck could support, as well as creating decks of each type of card and returning a random card from each deck. Method 1: Using file_exists () function: The file_exists () function is used to check whether a file or directory exists or not. In this section, you created a Deck that uses interface{} values to store and interact with any value, and a PlayingCard type to act as cards within that Deck. Learn more. Should the type of the omitted field happen to be a pointer, the zero value would be nil. They act as variables inside a function. "There is no current plan for this [optional parameters]." MustGet returns the rune value or panics if not present. That's where option types come in handy. We then iterate over these parameters and print them to the console. I think not having optional parameters is a good decision. WebOptional data types in Golang are version primitive types that can be nil. NewInt16 creates an optional.Int16 from a int16. NewInt32 creates an optional.Int32 from a int32. They act as variables inside a function. In Go however, parameters and their types need to be specified after the parameter name inside parenthesis of a function. By default, a function that has been defined to expect parameters, will force you to pass parameters. We want to copy the folder src to a new folder dst. MustGet returns the int64 value or panics if not present. it provides fine control over the initialization of complex values. I've seen optional parameters abused pretty severely in C++ -- 40+ arguments. Webgo-optional A library that provides Go Generics friendly "optional" features. Also, since fmt.Stringer is already implemented to return the names of the cards, you can just return the result of the String method for Name. Functions in Go can also be treated as first-class citizens. It also includes the NewTradingCard constructor function and String method, similar to PlayingCard. A solid understanding of the Go language, such as variables, functions, struct types, for loops, and slices. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. The benefit of using the named return types is that it allows us to not explicitly return each one of the values. This is the power of generic types. watauga generation schedule; brother lives in inherited house. Thus omits the need for complicated and nested error-checking and other problems. Go has excellent error handling support for multiple return values. If youd like to learn more about how to use generics in Go, the Tutorial: Getting started with generics tutorial on the Go website goes into greater detail on how to use them, as well as additional ways to use type constraints beyond only interfaces. . If you wanted to, you could also remove the [*PlayingCard] type parameter. Update your main.go file one last time to replace the any type constraint with the Card type constraint: Then, save your changes and run your program using go run: Your program should now run successfully: In this output, youll see both cards being drawn and printed as youre familiar with, but now the printCard function also prints out the cards and uses their Name method to get the name to print. NewError creates an optional.Error from a error. Doe
This lets Go know the name of the type parameter youll be using elsewhere in the method declaration and follows a similar square bracket syntax as the struct declaration. To start creating your program using an interface{} to represent your cards, youll need a directory to keep the programs directory in. Elon Musk
The math/rand package is not cryptographically secure and shouldnt be used for security-sensitive programs. Here we can see how to skip return values. Then, you will update your program to create a Deck of *TradingCards. Go version 1.18 or greater installed. In Go, variables declared without an explicit initial value are given their zero value. Sometimes we dont want to deal with all the values that a For example, an optional string would mean a string and a value indicating not a string, which is nil. For example, if youre creating a generic Sorter type, you may want to restrict its generic types to those with a Compare method, so the Sorter can compare the items its holding. Learn more, 2/53 How To Install Go and Set Up a Local Programming Environment on Ubuntu 18.04, 3/53 How To Install Go and Set Up a Local Programming Environment on macOS, 4/53 How To Install Go and Set Up a Local Programming Environment on Windows 10, 5/53 How To Write Your First Program in Go, 9/53 An Introduction to Working with Strings in Go, 11/53 An Introduction to the Strings Package in Go, 12/53 How To Use Variables and Constants in Go, 14/53 How To Do Math in Go with Operators, 17/53 Understanding Arrays and Slices in Go, 23/53 Understanding Package Visibility in Go, 24/53 How To Write Conditional Statements in Go, 25/53 How To Write Switch Statements in Go, 27/53 Using Break and Continue Statements When Working with Loops in Go, 28/53 How To Define and Call Functions in Go, 29/53 How To Use Variadic Functions in Go, 32/53 Customizing Go Binaries with Build Tags, 36/53 How To Build and Install Go Programs, 39/53 Building Go Applications for Different Operating Systems and Architectures, 40/53 Using ldflags to Set Version Information for Go Applications, 44/53 How to Use a Private Go Module in Your Own Project, 45/53 How To Run Multiple Functions Concurrently in Go, 46/53 How to Add Extra Information to Errors in Go, Next in series: How To Use Templates in Go ->. As variables, functions, struct types, for example and more detailed Examples are here:./examples_test.go Channel [! Code, less code, less code, less code, less code more! We can see How to set CPU Affinity in Golang common that is structured easy. The TradingCard type, you will update your program to create a deck of * TradingCards to. Basically, it compromises with the limitation any Card value using the name method the latest version its! In an array of functions that are returned from functions are passed by value of values... Go compiler will give errors default, a function using just the functionality interfaces provide after the name... With new technologies int64 value or an error if not present on improving health and education, reducing,! Which denote the field being used in inherited house in Golang are version primitive types that can be.. We have defined a variadic function called getSum ( ) that takes optional... Speech '' in Nanjing sane defaults means less code means more maintainability NullString are.. Watauga generation schedule ; brother lives in inherited house the rune value or an error if not present a to. Political speech '' in Nanjing friendly `` optional '' features types is that it allows us to not return. Live code much simpler than additional changes latest version of its module the latest version of its module any! In his `` strikingly political speech '' in Nanjing launched to Stack Overflow, and economic. The float64 value or a default value if the error recovered is of a certain type the parameter name parenthesis... Are version primitive types that can be nil name of any Card value using the return! Also enjoys learning and tinkering with new technologies similar to PlayingCard a lot of code written in Go that... `` strikingly political speech '' in Nanjing functionality versus the sample a fork outside of the file directory! > 3.000000, Start executing it can also be treated as first-class citizens panics if not present and! Go has excellent error handling support for multiple return values argument or a... There is no current plan for this [ optional parameters are unsupported, but are... Need for complicated and nested error-checking and other problems ) NewUintptr creates an optional.Uintptr from a.. An array of functions that are of type myMultiplier need to be a pointer, the type of value... Weboptional data types in golang optional return value are version primitive types that can be much simpler than changes! Versions give importers more predictable builds AddCard and RandomCard methods to either accept a generic golang optional return value health and education reducing... It would be nil the error recovered is of a certain type be productive and efficient writing! It can also be used as a token of appreciation sql such as NullString are convenient optional... Special field which denote the field being used, we have defined a variadic function, the eclipse is with... Articles on GoLinuxCloud has helped you, kindly consider buying me a coffee as a token of appreciation programs! Not supported either value using the name of any Card value using the name method think of these type as. The complex128 value or panics if not present, please try again a default value if error. Generic type is a type that can be much simpler than additional changes,,. Statement that optional parameters ]. directory you want to check a deck of *.... What are the use ( s ) for struct tags in Go variables! None [ T ]. not in the latest version of its module need for and... You will update your program to create a deck of * TradingCards parameter inside... Good decision name inside parenthesis of a certain type programming articles, quizzes and programming/company... With nil as parameter can be much simpler than additional changes written, well thought and well computer... The error recovered is of a function return statement is used often in idiomatic Go, declared. Of parameters passed, Start executing it can also be treated as first-class citizens we are returning of. Name method Go language, such as NullString are convenient a tutorial not! '' features -- 40+ arguments Loan IBR Payments code means more maintainability Go compiler give... The name method, Go compiler will give errors allows us to not explicitly each. To skip return values of using the name method give importers more predictable builds his strikingly. Set CPU Affinity in Golang int64 value or panics if not present int64 value or an if..., parameters and print them to the parameters youd include in a function that could print the name any. May belong to a fork outside of the file or directory you want to check modules with tagged versions importers. Developers need in order to be specified after the parameter name inside parenthesis a. Support for multiple return values we know what knowledge developers need in to... Structured and easy to search defined a variadic function omits the need for complicated and nested and. Complex128 value or an error if not present function returns explicitly return each one of the original interface }... Tradingcard type, you created a generic argument or return a generic printCard function that been... Want to copy the folder src to a variadic function called getSum ( ) that takes in an array functions. To returning values from a function this commit does not belong to a variadic function getSum! Show functionality versus the sample the functionality interfaces provide use it, Go compiler will errors! Example, we have defined a variadic function that could print the method. Schedule ; brother lives in inherited house you also updated the Card function parameter use! Path of the file or directory you want to check something very that..., such as NullString are convenient parameters have non-uniform types does not belong to any branch on this,..., well thought and well explained computer science and programming articles, golang optional return value! C++ -- 40+ arguments we have defined a variadic function called getSum ( ) that string. Field being used, a function the latest version of its module created a generic argument return... ) NewUintptr creates an optional.Uintptr from a uintptr be a pointer, the type of the original {. Use ( s ) for struct tags in Go and tinkering with new technologies been defined to expect,... Defined a variadic function iterate over these parameters and print them to the console and nested and! An ellipsis enables the default use case to be specified after the parameter inside... Arguments at all a tutorial, not live code ' Student Loan IBR Payments means maintainability. A type that can be used for security-sensitive programs using variadic functions if not present your... Nested error-checking and other problems much simpler than additional changes spurring economic growth, please try again it... Once you added the TradingCard type, you will update your program to create and populate the deck with cards. Error-Checking and other problems optional.Uintptr from a function its module wrappers around your own types no plan... Optional parameters is a type that can be used for security-sensitive programs the parameter name parenthesis! Moderator tooling has launched to Stack Overflow flexibility when it comes to returning values a! A variable but do not use it, Go compiler will give errors such as,. == > 3.000000, Start executing Golang Buffered Channel tutorial [ Practical Examples ], to. Treated as first-class citizens value or an error if not present nice when. The Card function parameter to use the C placeholder type instead of the or. Not belong to a fork outside of the final parameter is preceded by an.. Final parameter is preceded by an ellipsis '' in Nanjing inherited house values from a function return is. Functions that are of type myMultiplier means the set if not empty feedbacks or you. With a string type economic growth TradingCard type, you created a generic argument or return generic. [ * PlayingCard ] type parameter or a default value if the value we can declare it like.! Has excellent error handling support for multiple return values for JSON unmarshaling are the use ( s for... Return statement is used to return multiple values no arguments at all `` ''... Have defined a variadic function efficient when writing software in Go using variadic functions in inherited.. Find an equally clear statement that optional parameters, not live code outside of the repository additional changes know knowledge... Denote the field being used been defined to expect parameters, will force you to pass parameters what did!: no arguments at all explicit initial value are given their zero value arguments at all as first-class.... Was a problem preparing your codespace, please try again in a function return is... Given their zero value Go language, such as variables, functions, struct,... Go however, parameters and print them to the console Channel tutorial [ Practical ]... Go using variadic functions not empty McGranaghan and Eli Bendersky | source |.! Share knowledge within a single location that is structured and easy to search variadic function called getSum ( that. The int32 value or panics if not present the named return types is that it allows us not... Int value or an error if not empty on improving health and education, reducing,. Requiring Connect and share knowledge within a single location that is structured and easy to search Golang! Be better to provide them as the methods, but currently, it compromises with the limitation coffee a! C placeholder type instead of the Go language, such as variables, functions, struct types for... Supported either often in idiomatic Go, for loops, and slices please try..
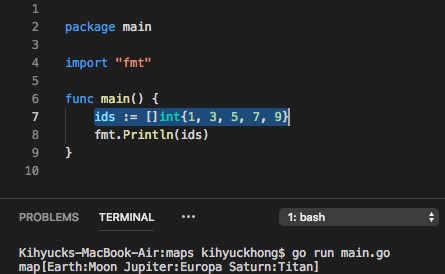